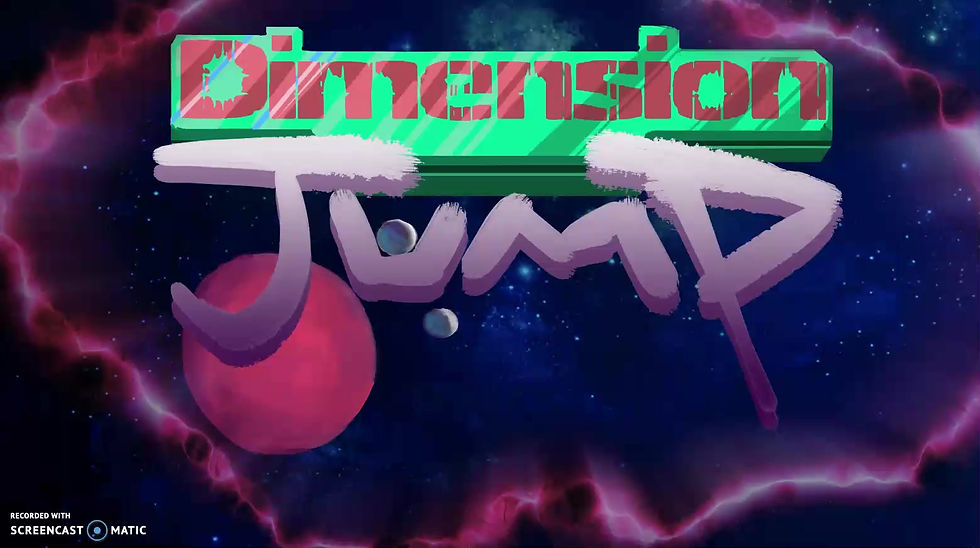
DIMENSION JUMP
Now you're thinking with portals
Dimension Jump
Press Play
Dimension Jump is a fast paced 2d side scrolling platformer that follows our fun loving hero Amose chasing his mysterious enemy on a colourful sci-fi puzzle adventure. To progress Amose must learn to use his his dimension Jumping Power to solve puzzles based on memory, timing and precision that involve quickly switching dimensions while on the move and finding the correct path. I worked on this project as a programmer, animator and a level designer.
Main Character Animations
View Now
Main Character Movemnt
View Now
Main Game Mechanic
Have a Look
Main Character Shoot Mechanic
Have a Look
Turret Enemy with homing bullets
View Now
Main Game Mechanic
-
This Mechanic works by first by placing the script onto a empty gameobject.
-
Then you place all the unique assets of each of the environments into their own group.
-
Then in your script you create variables for both groups, and in my case both TileMaps, and connect them appropriately in the inspecter to the gameobject with script.
-
Then you use code like this:
​
public class AbilityControllerV2 : MonoBehaviour {
bool blueTrue;
public LevelManager theLM;
public GameObject B;
public GameObject R;
public GameObject egypt;
public GameObject Strange;
​
void Awake()
{
blueTrue = false;
B.SetActive (false);
LayerSwitch();
Strange.SetActive (false);
}
​
public void LayerSwitch()
{
blueTrue = !blueTrue;
​
if(blueTrue == false)
{
R.SetActive (false);
B.SetActive (true);
Strange.SetActive (true);
egypt.SetActive (false);
}
​
if(blueTrue == true)
{
R.SetActive (true);
egypt.SetActive (true);
B.SetActive (false);
Strange.SetActive (false);
}
}
}
​
5.Now if you set a input to call LayerSwitch it will switch out the two groups.
Homing Bullet Mechanic
-
To make bullets home the way I did it you place a large box collider around your enemy or turret to act as the range.
-
You also need a empty game object that is parented to your enemy , mine is called target, and connect it to the enemy in the inspector.
-
Have a variable for your bullet and put your bullets prefab into it in the inspector.
-
Then you use code like this:
​
public class EnemyV2Script : MonoBehaviour {
public AudioScript audioScript;
public GameObject theHero;
public Transform gunPos;
public GameObject bO;
public GameObject target;
bool facingRight = true;
Animator anim;
public LevelManager theLM;
public bool fireOn;
public Transform theTarget;
GameObject aBullet;
void Start ()
{
anim = GetComponent<Animator>();
theLM = GameObject.FindGameObjectWithTag("LM").GetComponent<LevelManager>();
theHero = GameObject.FindGameObjectWithTag("Player");
audioScript = GameObject.FindGameObjectWithTag("Audio").GetComponent<AudioScript>();
fireOn = false;
}
void Update ()
{
}
public void OnTriggerEnter2D(Collider2D collision)
{
if (collision.gameObject.tag.Equals("Player"))
{
fireOn = true;
Shoot();
}
}
public void OnTriggerExit2D(Collider2D collision)
{
if (collision.gameObject.tag.Equals("Player"))
{
fireOn = false;
target.transform.position = gameObject.transform.position;
target.transform.parent = gameObject.transform;
Destroy(aBullet);
}
}
public void FixedUpdate()
{
if(fireOn == true)
{
target.transform.position = theHero.transform.position;
target.transform.parent = theHero.transform;
aBullet.transform.position = Vector3.MoveTowards(aBullet.transform.position, target.transform.position, 0.1f);
}
}
void Flip()
{
facingRight = !facingRight;
Vector3 theScale = transform.localScale;
theScale.x *= -1;
transform.localScale = theScale;
}
public void Shoot()
{
anim.SetTrigger("Shoot");
aBullet = Instantiate(bO, gunPos.position, gunPos.rotation);
audioScript.enemyShotSX();
}
}
​
4. The bullet will move straight towards the target gameobject that is not on the hero and is now a child of the hero.